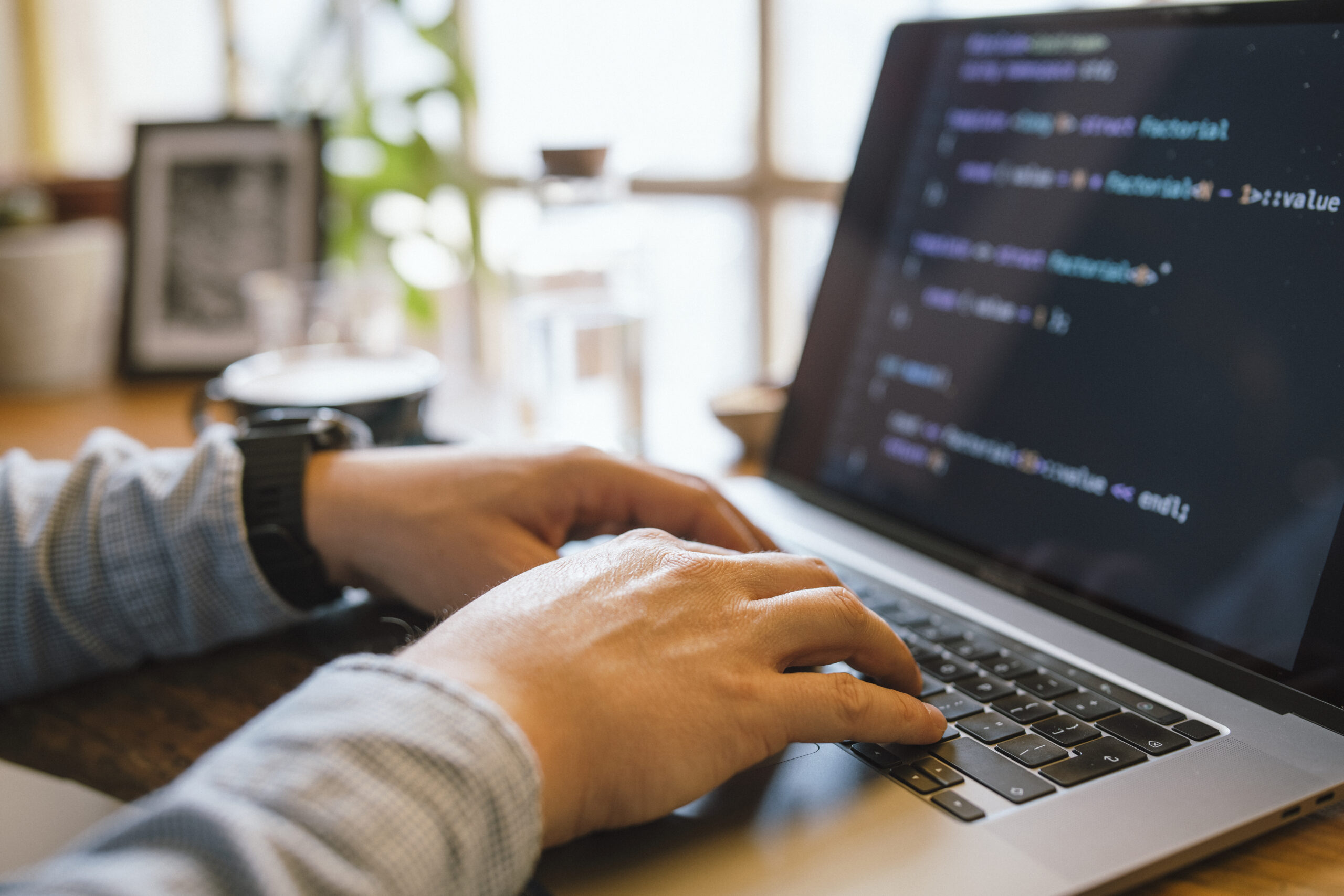
Debugging is Just about the most necessary — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It isn't nearly correcting damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Imagine methodically to unravel difficulties proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging capabilities can save hours of frustration and significantly enhance your productivity. Here i will discuss quite a few procedures to help developers degree up their debugging sport by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest means builders can elevate their debugging capabilities is by mastering the resources they use daily. Whilst creating code is one Component of enhancement, figuring out tips on how to communicate with it properly throughout execution is Similarly significant. Modern day improvement environments occur Outfitted with highly effective debugging capabilities — but numerous developers only scratch the floor of what these instruments can do.
Choose, such as, an Built-in Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources help you set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, and in many cases modify code about the fly. When applied appropriately, they let you observe exactly how your code behaves for the duration of execution, that is invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can flip discouraging UI problems into workable responsibilities.
For backend or program-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate over working procedures and memory management. Finding out these tools might have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, grow to be at ease with Variation Manage techniques like Git to be aware of code record, discover the precise moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications signifies going past default options and shortcuts — it’s about establishing an personal familiarity with your progress surroundings making sure that when challenges arise, you’re not misplaced at nighttime. The higher you recognize your applications, the more time you can invest solving the actual problem as opposed to fumbling by way of the method.
Reproduce the trouble
The most essential — and sometimes disregarded — ways in helpful debugging is reproducing the situation. In advance of leaping to the code or creating guesses, developers want to create a dependable ecosystem or situation where by the bug reliably seems. Devoid of reproducibility, correcting a bug results in being a video game of likelihood, frequently bringing about squandered time and fragile code adjustments.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire questions like: What steps triggered The problem? Which atmosphere was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've got, the simpler it results in being to isolate the precise situations under which the bug takes place.
As soon as you’ve gathered plenty of details, try to recreate the situation in your local ecosystem. This may suggest inputting the same facts, simulating comparable consumer interactions, or mimicking method states. If The problem seems intermittently, take into consideration producing automated exams that replicate the sting cases or condition transitions associated. These assessments not only support expose the condition but in addition protect against regressions in the future.
Often, the issue can be environment-certain — it'd happen only on specified functioning systems, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating this sort of bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, along with a methodical technique. But once you can constantly recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should utilize your debugging applications extra effectively, test possible fixes securely, and talk far more Evidently with all your team or users. It turns an summary criticism right into a concrete obstacle — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most worthy clues a developer has when a little something goes Completely wrong. Rather then observing them as annoying interruptions, developers must find out to deal with error messages as direct communications within the process. They generally inform you just what happened, the place it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start out by looking through the concept very carefully and in complete. Numerous builders, particularly when below time tension, look at the primary line and instantly start building assumptions. But deeper in the mistake stack or logs may perhaps lie the genuine root induce. Don’t just duplicate and paste mistake messages into search engines — examine and understand them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or simply a logic error? Does it issue to a particular file and line number? What module or functionality induced it? These questions can tutorial your investigation and stage you toward the responsible code.
It’s also practical to comprehend the terminology of your programming language or framework you’re employing. Error messages in languages like Python, JavaScript, or Java usually follow predictable patterns, and Mastering to recognize these can drastically quicken your debugging system.
Some mistakes are obscure or generic, As well as in Those people circumstances, it’s very important to examine the context through which the mistake occurred. Examine linked log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger issues and provide hints about opportunity bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, aiding you pinpoint troubles a lot quicker, lessen debugging time, and turn into a extra efficient and assured developer.
Use Logging Correctly
Logging is one of the most effective instruments within a developer’s debugging toolkit. When applied correctly, it offers real-time insights into how an application behaves, helping you comprehend what’s taking place beneath the hood without having to pause execution or move in the code line by line.
A good logging technique begins with realizing what to log and at what degree. Widespread logging stages involve DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for click here typical gatherings (like prosperous start off-ups), WARN for possible problems that don’t break the applying, Mistake for precise challenges, and Lethal once the method can’t carry on.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure vital messages and decelerate your method. Focus on vital gatherings, condition modifications, enter/output values, and significant final decision points in your code.
Structure your log messages clearly and continually. Incorporate context, like timestamps, ask for IDs, and function names, so it’s easier to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re especially precious in manufacturing environments wherever stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-assumed-out logging strategy, you could decrease the time it takes to spot difficulties, gain deeper visibility into your purposes, and improve the Total maintainability and trustworthiness of the code.
Feel Similar to a Detective
Debugging is not just a specialized undertaking—it is a type of investigation. To properly identify and resolve bugs, builders need to solution the process just like a detective fixing a secret. This state of mind will help stop working complex difficulties into manageable components and follow clues logically to uncover the root cause.
Start by gathering evidence. Look at the indicators of the issue: error messages, incorrect output, or general performance concerns. Similar to a detective surveys a crime scene, obtain as much relevant details as it is possible to devoid of jumping to conclusions. Use logs, take a look at instances, and user studies to piece collectively a transparent photograph of what’s going on.
Upcoming, sort hypotheses. Check with on your own: What may very well be creating this habits? Have any alterations not long ago been designed on the codebase? Has this issue happened in advance of underneath related conditions? The aim is always to narrow down possibilities and detect possible culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Pay out shut consideration to smaller specifics. Bugs often cover inside the the very least predicted places—similar to a missing semicolon, an off-by-a person error, or even a race ailment. Be extensive and patient, resisting the urge to patch The problem with out thoroughly knowing it. Non permanent fixes could disguise the real problem, only for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term challenges and aid Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and turn into more practical at uncovering hidden challenges in sophisticated units.
Create Exams
Producing checks is one of the most effective strategies to help your debugging skills and Over-all enhancement efficiency. Tests not just support capture bugs early and also function a security Web that offers you self confidence when earning changes to your codebase. A nicely-tested application is easier to debug since it permits you to pinpoint just where by and when a problem happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated exams can rapidly reveal whether or not a particular bit of logic is Doing work as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing the time spent debugging. Device tests are especially practical for catching regression bugs—difficulties that reappear immediately after Earlier getting set.
Subsequent, combine integration assessments and conclude-to-stop tests into your workflow. These assist make sure that various aspects of your software perform together easily. They’re especially practical for catching bugs that come about in sophisticated methods with a number of components or products and services interacting. If some thing breaks, your assessments can let you know which part of the pipeline unsuccessful and beneath what situations.
Crafting assessments also forces you to definitely Consider critically about your code. To check a feature thoroughly, you require to comprehend its inputs, envisioned outputs, and edge situations. This level of knowledge Normally potential customers to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug might be a robust first step. When the test fails persistently, you can target fixing the bug and look at your test pass when The difficulty is resolved. This technique makes certain that the identical bug doesn’t return Down the road.
In short, composing assessments turns debugging from the irritating guessing game into a structured and predictable course of action—encouraging you capture far more bugs, more quickly plus more reliably.
Consider Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—watching your display screen for several hours, trying Remedy soon after Option. But one of the most underrated debugging resources is just stepping away. Using breaks will help you reset your head, decrease stress, and sometimes see The problem from the new point of view.
When you are way too near to the code for far too very long, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable mistakes or misreading code that you simply wrote just hours earlier. In this state, your Mind results in being fewer economical at trouble-resolving. A short walk, a coffee crack, or perhaps switching to a special task for ten–quarter-hour can refresh your concentration. Many developers report locating the root of a problem when they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also enable avoid burnout, Specifically throughout longer debugging classes. Sitting in front of a display screen, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Vitality along with a clearer mentality. You could possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
When you’re stuck, a very good guideline is to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than restricted deadlines, but it really truly results in speedier and more effective debugging In the long term.
In short, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Learn From Each and every Bug
Just about every bug you experience is much more than simply a temporary setback—It really is a possibility to mature for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can educate you anything important in the event you make time to replicate and review what went wrong.
Begin by asking by yourself some key inquiries when the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with much better procedures like unit screening, code testimonials, or logging? The solutions typically expose blind places with your workflow or knowledge and assist you Establish more powerful coding behavior relocating forward.
Documenting bugs may also be a superb behavior. Preserve a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see styles—recurring difficulties or widespread problems—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends might be Specifically potent. Whether it’s via a Slack concept, a short generate-up, or A fast information-sharing session, assisting Other people steer clear of the same difficulty boosts staff efficiency and cultivates a stronger Mastering tradition.
Much more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Instead of dreading bugs, you’ll start off appreciating them as crucial aspects of your growth journey. In the end, a lot of the ideal developers are certainly not those who write best code, but people who consistently find out from their issues.
Ultimately, each bug you correct provides a fresh layer towards your skill established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, much more able developer as a result of it.
Summary
Improving your debugging capabilities takes time, follow, and endurance — but the payoff is big. It would make you a more effective, self-confident, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.